interface는 클래스 또는 객체를 위한 타입을 지정 할 때 사용되는 문법입니다.
우리가 클래스를 만들 때, 특정 조건을 준수해야 함을 명시하고 싶을 때 interface를 사용하여 클래스가 가지고 있어야 할 요구사항을 설정합니다. 그리고 클래스를 선언 할 때 implements키워드를 사용하여 해당 클래스가 특정 interface의 요구사항을 구현한다는 것을 명시합니다.
다음 코드를 작성해봅시다.
// Shape 라는 interface 를 선언
interface Shape {
getArea(): number;
// Shape interface 에는 getArea 라는 함수가 꼭 있어야 하며 해당 함수의 반환값은 number입니다.
}
class Circle implements Shape {
// `implements` 키워드를 사용하여 해당 클래스가 Shape interface 의 조건을 충족하겠다는 것을 명시합니다.
radius: number; // 멤버 변수 number타입의 radius값을 설정합니다.
//생성자
constructor(radius: number) {
this.radius = radius;
}
// 너비를 가져오는 함수를 구현합니다.
getArea() {
return this.radius * this.radius * Math.PI;
}
}
class Rectangle implements Shape {
width: number;
height: number;
constructor(width: number, height: number) {
this.width = width;
this.height = height;
}
getArea() {
return this.width * this.height;
}
}
const shapes: Shape[] = [new Circle(5), new Rectangle(10, 5)];
shapes.forEach(shape => {
console.log(shape.getArea());
});
코드를 작성하고 디렉토리에서 tsc 명령어를 입력해 컴파일을 해봅시다.
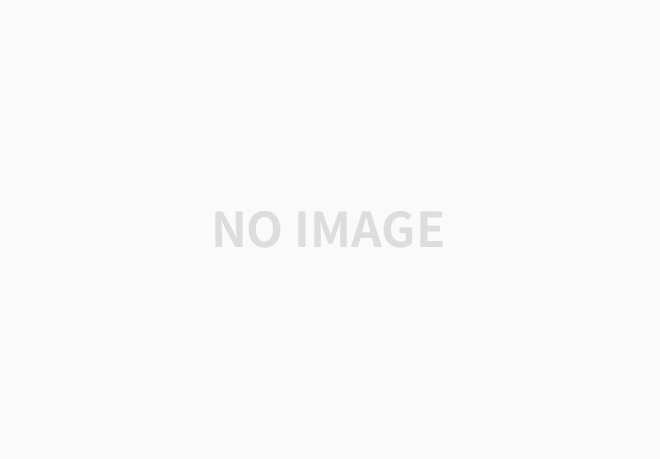
./dist 디렉토리에 컴파일된 js파일이 생성되었습니다.
실행해 볼까요?
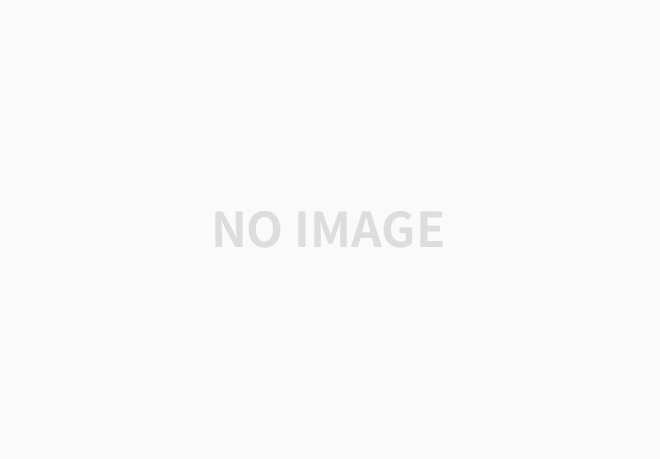
잘 작동됩니다.
위에서 작성했던 코드에서 생성자(constructor) 부분을 보면
width: number;
height: number;
constructor(width: number, height: number) {
this.width = width;
this.height = height;
}
이런식으로 width, height 멤버 변수를 선언한 다음에 constructor 에서 해당 값들을 하나 하나 설정해주었는데 타입스크립트에서는 constructor 의 파라미터 쪽에 public 또는 private를 사용하면 직접 하나하나 설정해주는 작업을 생략해줄 수 있습니다.
interface Shape {
getArea(): number;
}
class Circle implements Shape {
//radius: number;
constructor(public radius: number){
this.radius = radius;
}
getArea(){
return this.radius * this.radius * Math.PI;
}
}
class Rectangle implements Shape {
//width: number;
//height : number;
constructor(private width: number, private height: number){
this.width = width;
this.height = height;
}
getArea(){
return this.width * this.height;
}
}
const circle = new Circle(5);
const rectangle = new Rectangle(10, 5);
console.log(circle.radius);
//console.log(rectangle.width); width 멤버 변수는 private이기 때문에 밖에서 조회 불가능
const shapes: Shape[] = [new Circle(5), new Rectangle(10, 5)];
shapes.forEach(shape => {
console.log(shape.getArea());
});
일반 객체를 interface 로 타입 설정하기
일반 객체를 interface를 사용하여 타입을 지정하는 방법을 알아보도록 하겠습니다.
interface Person {
name: string;
age?: number; // 물음표가 들어갔다는 것은, 설정을 해도 되고 안해도 되는 값이라는 것을 의미
}
interface Developer {
name: string;
age?: number;
skills: string[];
}
const person: Person = {
name: '김사람',
age: 20
};
const expert: Developer = {
name: '김개발',
skills: ['javascript', 'react']
};
Person과 Developer가 형태가 유사한데 이럴 때 interface를 선언 할 때 다른 interface를 extends해서 상속받을 수 있습니다.
interface Person {
name: string;
age?: number; // 물음표가 들어갔다는 것은, 설정을 해도 되고 안해도 되는 값이라는 것을 의미
}
interface Developer extends Person //Person의 name, age는 상속됨.
skills: string[]; //따라서 새로운 멤버 변수 skills만 추가해주면됨.
}
const person: Person = {
name: '김사람',
age: 20
};
const expert: Developer = {
name: '김개발',
skills: ['javascript', 'react']
};
const people: Person[] = [person, expert];
Type Alias 사용하기
type은 특정 타입에 별칭을 붙이는 용도로 사용합니다. 이를 사용하여 객체를 위한 타입을 설정할 수도 있고, 배열, 또는 그 어떤 타입이던 별칭을 지어줄 수 있습니다.
type Person = {
name: string;
age?: number; // 물음표가 들어갔다는 것은, 설정을 해도 되고 안해도 되는 값이라는 것을 의미합니다.
};
// & 는 Intersection 으로서 두개 이상의 타입들을 합쳐줍니다.
// 참고: https://www.typescriptlang.org/docs/handbook/advanced-types.html#intersection-types
type Developer = Person & {
skills: string[];
};
const person: Person = {
name: '김사람'
};
const expert: Developer = {
name: '김개발',
skills: ['javascript', 'react']
};
type People = Person[]; // Person[] 를 이제 앞으로 People 이라는 타입으로 사용 할 수 있습니다.
const people: People = [person, expert];
type Color = 'red' | 'orange' | 'yellow';
const color: Color = 'red';
const colors: Color[] = ['red', 'orange'];
이번에 type과 interface를 배웠는데, 어떤 용도로 사용을 해야 할까요? 무엇이든 써도 상관 없는데 일관성 있게만 쓰시면 됩니다. 구버전의 타입스크립트에서는 type과 interface의 차이가 많이 존재했었는데 이제는 큰 차이는 없습니다. 다만 라이브러리를 작성하거나 다른 라이브러리를 위한 타입 지원 파일을 작성하게 될 때는 interface를 사용하는것이 권장 되고 있습니다.
'programming_kr > typescript' 카테고리의 다른 글
tsconfig.json "구성 파일에서 입력을 찾을 수 없습니다." 오류 (1) | 2021.10.22 |
---|---|
Type Script 기초 배우기 5[Generics] (0) | 2021.10.14 |
Type Script 기초 배우기 3[함수] (0) | 2021.10.08 |
Type Script 기초 배우기 2[변수] (0) | 2021.10.05 |
Type Script 기초 배우기 1 (0) | 2021.10.02 |
댓글